Content Audit
Premium Feature
This feature is only available in Growth and Enterprise plans
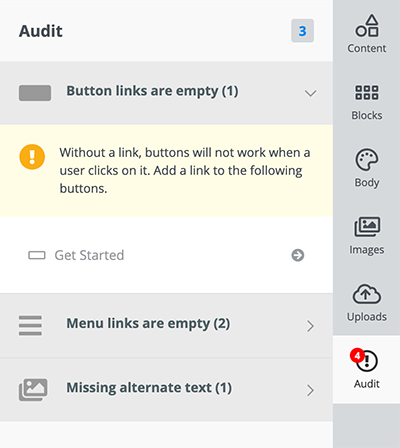
The Audit tab flags common issues so you can address them before finishing your email or page design. It currently looks for accessibility checks so you can identify and fix common issues during the design process. It addresses:
- Missing alternate text for images
- Missing links in buttons or menus
- Missing image URLs
As an added bonus, by using the Audit tab and learning from the issues it flags, you can become more practiced and aware of these considerations so they’re part of your design and development workflow instead of an afterthought.
Enable / Disable
You can enable or disable this feature.
unlayer.init({
features: {
audit: true
}
})
Custom Validators
If you want to run the content for other types of issues, you can create custom validators.
Global Validator
A global validator can be used to identify general design issues. For example, if you want to run and analyze the content for potential spamminess.
unlayer.setValidator(function (data) {
const { html, design, defaultErrors } = data;
return [
{
id: 'DESIGN_CUSTOM_ERROR',
icon: 'fa-smile',
severity: 'WARNING',
title: 'This is a custom error',
description:
'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Fusce pretium non lectus venenatis lacinia. Fusce vitae venenatis nibh. Sed laoreet ornare lorem, a convallis nibh suscipit eu. Ut dictum commodo velit vitae interdum.',
},
].concat(defaultErrors);
});
Each error needs to have the following parameters.
Name | Description |
---|---|
id | A unique ID for the error type |
icon | Icon that users see in the errors tab. The value can be class name of any fontawesome icon, or a URL for your icon's image |
severity | ERROR or WARNING |
title | Title of the error message |
description | Description of the error message |
Tool Validator
Similar to the global validator, you can also create custom validators for different tools. This can be done for built-in tools, as well as custom tools, using 'custom#tool_name' as the first argument. These validators can check and validate different values of the tools' properties.
Here's an example of attaching a validator to the button
tool.
unlayer.setToolValidator('button', function(data) {
const { defaultErrors, values } = data;
return [
{
id: 'CUSTOM_ERROR',
icon: 'fa-smile',
severity: 'WARNING',
title: 'This is a custom button error',
description: `Lorem ipsum dolor sit amet ${values?.text}.`,
},
].concat(defaultErrors);
});
Translations (i18n) for Validators
The title and description for each error can include translated text to keep it i18n compliant. You can read more about our localization.
unlayer.init({
translations: {
en: {
custom_translation_title: 'This is a custom title',
custom_translation_desc: 'This is a custom description.',
},
},
});
unlayer.setToolValidator('button', function (data) {
return [
{
id: 'CUSTOM_ERROR',
icon: 'fa-smile',
severity: 'WARNING',
title: 'custom_translation_title',
description: 'custom_translation_desc',
},
];
});
Check Status
You can check if there are any active errors or warnings in the design by using the following method.
unlayer.audit(function(data) {
console.log(data);
// {
// status: "PASS",
// errors: [],
// }
// {
// status: "FAIL",
// errors: [{...}],
// }
});
Spam Example
Here's an async example where you can use an external API to check your content for spam.
unlayer.setValidator(async (data) => {
const { html, design, defaultErrors } = data
const errors = []
// Check HTML for spamminess using a third-party API
const isSpammy = await isHtmlSpammy(html);
if (isSpammy) {
errors.push(
{
id: 'SPAM_ERROR',
icon: 'fa-image',
severity: 'WARNING',
title: 'Content is Spammy',
description:
'The content seems to be spammy. Fix your content.',
}
);
}
return errors.concat(defaultErrors);
});
Updated over 1 year ago