User Uploads
Premium Feature
This feature is only available in premium plans. You must pass the
projectId
with a paid plan to enable this feature.
We have a Uploads tab that stores all user uploaded images so your users can access their uploaded images at any point.
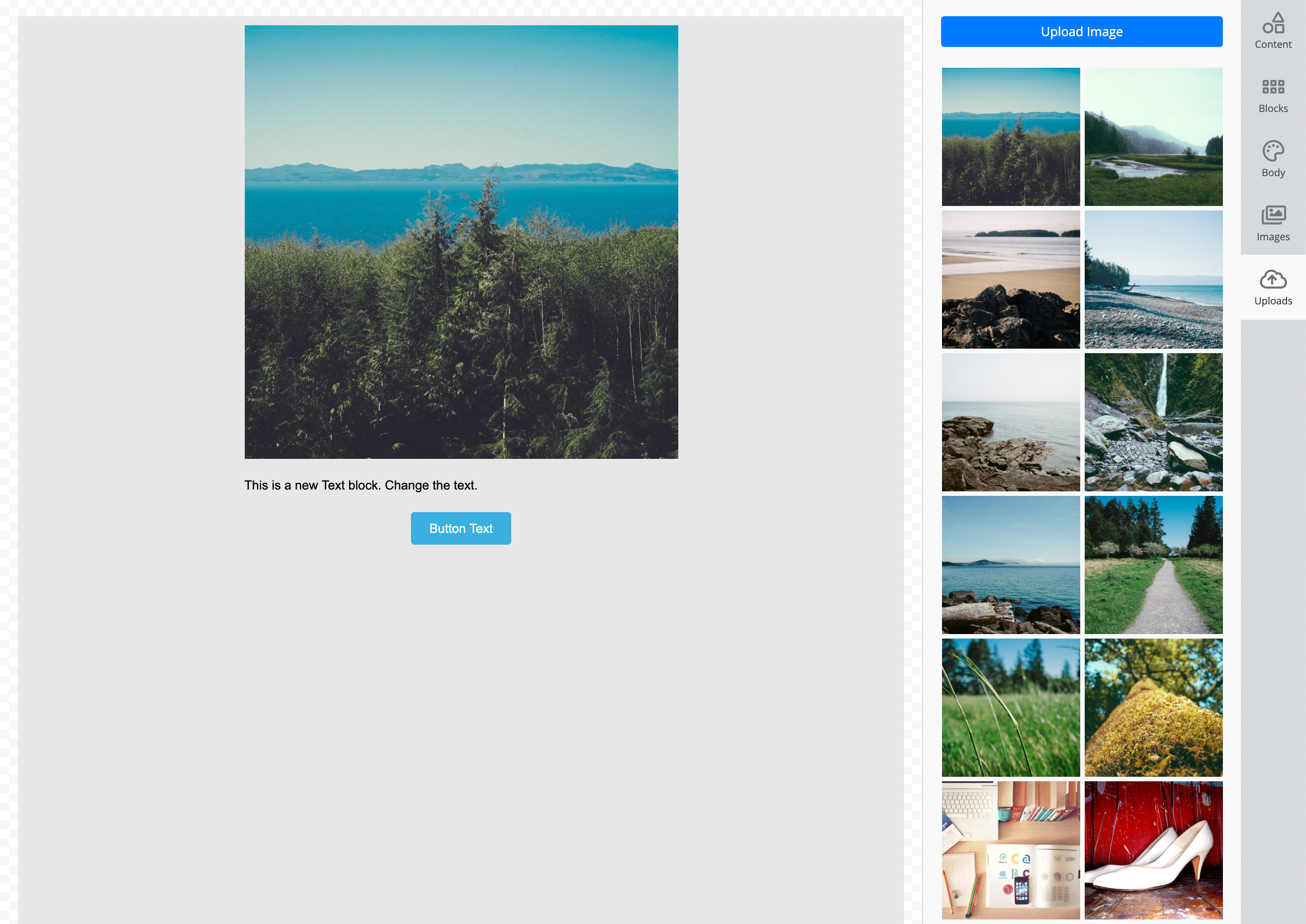
Enable / Disable Feature
You can enable the uploads tab by passing a user object with the unique user id. The user object must have a unique user id for the user who is using the editor. The id can be a number or a string.
unlayer.init({
user: {
id: 1
}
})
If you want to disable the uploads tab, use the following:
unlayer.init({
features: {
userUploads: false
}
})
Custom Database
If your users' images are stored in your database already and you want the uploads tab to show those images, use the following JavaScript API. Each image should have the following attributes.
Attribute | Description |
---|---|
id | Unique ID of the image |
location | URL of the image |
width | Width of the image |
height | Height of the image |
contentType | Content MIME type of the image |
source | This should always be user |
size | Optional. File size of the image |
You can check out a full example using custom database to load user uploaded images.
unlayer.registerProvider('userUploads', function (params, done) {
// Load images from your server here...
var images = [
{
id: Date.now() + i,
location: 'https://picsum.photos/id/1/500',
width: 500,
height: 500,
contentType: 'image/png',
source: 'user'
},
{
id: Date.now() + i,
location: 'https://picsum.photos/id/2/500',
width: 500,
height: 500,
contentType: 'image/png',
source: 'user'
}
];
done(images);
});
Pagination
You can utilize pagination features of the uploads tab. In additional to the images, you can provide the following pagination attributes.
Attribute | Description |
---|---|
page | Current page |
perPage | How many images to show on each page |
total | Total images in the database |
hasMore | Does the user have more images? If true, load more button will be available |
unlayer.registerProvider('userUploads', function (params, done) {
var page = params.page || 1;
var perPage = params.perPage || 20;
var total = 100;
var hasMore = total > page * perPage;
// Load images for page X from your server here...
var images = [];
done(images, { hasMore, page, perPage, total });
});
Handling Deletions
Since these are user uploaded images, the users have the ability to delete them as well. You can use the following callback to handle deletions in your application.
unlayer.registerCallback('image:removed', function (image, done) {
// image will include id, userId and projectId
console.log(image);
// call this when image has been deleted
done();
});
Updated over 3 years ago