Features
You can enable or disable certain features of the Unlayer editor. Most of these are enabled by default but can be turned off using the following configuration.
Mobile / Desktop Preview
You can choose to hide the mobile / desktop preview icons from the little actions box at the bottom.
unlayer.init({
features: {
preview: false
}
})
You can show or hide this preview programmatically as well.
// Show desktop preview
unlayer.showPreview("desktop");
// Show mobile preview
unlayer.showPreview("mobile");
// Hide the preview
unlayer.hidePreview();
If you want to control what to show in preview mode, you have the option pass custom HTML. This is especially useful if you want to parse your template with a templating library and show dynamic content.
unlayer.registerCallback('previewHtml', function (params, done) {
done({
html: params.html // you can pass your custom html here
});
});
Image Editor
Premium Feature
This feature is only available in premium plans
We have a built-in image editor that can be used to resize, crop or apply effects to images. It's enabled by default but you can disable it if you want.
unlayer.init({
features: {
imageEditor: {
enabled: false
}
}
})
Image Editor Tools
You can also choose to disable any of the image editor tools. By default, all of these tools are enabled: Filter, Resize, Crop, Draw, Text, Shapes, Stickers, Frame, Corners and Merge.
Here's an example if you want to disable the Resize tool in the image editor.
unlayer.init({
features: {
imageEditor: {
tools: {
resize: false
}
}
}
});
User Uploads
Premium Feature
This feature is only available in premium plans
We have a Uploads
tab that can show all uploaded images for the user. It's enabled by default but you can disable it if you want.
unlayer.init({
features: {
userUploads: false
}
})
Stock Images
Premium Feature
This feature is only available in premium plans
We have a built-in stock images library with millions of royalty-free images from Unsplash, Pexels and Pixabay. It's enabled by default but you can disable it if you want. You can also choose to change the safe searching option and the default search term.
unlayer.init({
features: {
stockImages: {
enabled: true,
safeSearch: true,
defaultSearchTerm: "people"
}
}
})
Audit Content
Premium Feature
This feature is only available in Growth and Enterprise plans
The Audit tab flags common accessibility-related issues so you can address them before finishing your design. It helps identify issues like missing links, missing alternate text, etc. You can enable or disable this feature.
unlayer.init({
features: {
audit: true
}
})
You can also add custom validators to the audit tab. Learn More
Page Anchors
This is a great feature for linking within a page. It lets users define sections of a page and then link buttons or links to those sections.
You can enable this using the following code.
unlayer.init({
features: {
pageAnchors: true
}
})
Undo / Redo
You can choose to hide the undo / redo buttons from the little actions box at the bottom. If you choose to hide the undo / redo buttons, you may want to turn on delete confirmation setting.
unlayer.init({
features: {
undoRedo: false
}
})
Undo and redo functions can also be called programmatically using the following code:
// Undo last action
unlayer.undo()
// Redo last action
unlayer.redo()
// Any action in queue to undo?
unlayer.canUndo()
// Any action in queue to redo?
unlayer.canRedo()
Text Editor
Spell Checker
You can enable or disable the browser spell checker for the text editor.
unlayer.init({
features: {
textEditor: {
spellChecker: true
}
}
})
Tables
You can enable or disable tables editing for the text editor.
unlayer.init({
features: {
textEditor: {
tables: true
}
}
})
Clean Paste
By default, clean pasting is set to confirm
. You can set it to any of the following values.
Value | Description |
---|---|
true | Clean pasting is turned on. No formatting will be added. |
false | Clean pasting is turned off. Formatting will be preserved. |
basic | Structure and only basic formatting is preserved. |
confirm (default) | Confirms from the user whether to keep all formatting or only basic formatting. |
unlayer.init({
features: {
textEditor: {
cleanPaste: false
}
}
})
Emojis
By default, emoticons are turned on in the text editor. If you want to disable it, you can turn this off.
unlayer.init({
features: {
textEditor: {
emojis: false
}
}
})
Inline Font Controls
You can enable or disable the inline font controls such as font family, font size, and text alignment. We do not recommend enabling this feature because these controls are available natively in the right panel and that works better with responsiveness across devices.
unlayer.init({
features: {
textEditor: {
inlineFontControls: false
}
}
})
Custom Buttons
You can add custom buttons to the text editor to build more advanced and powerful controls for text.
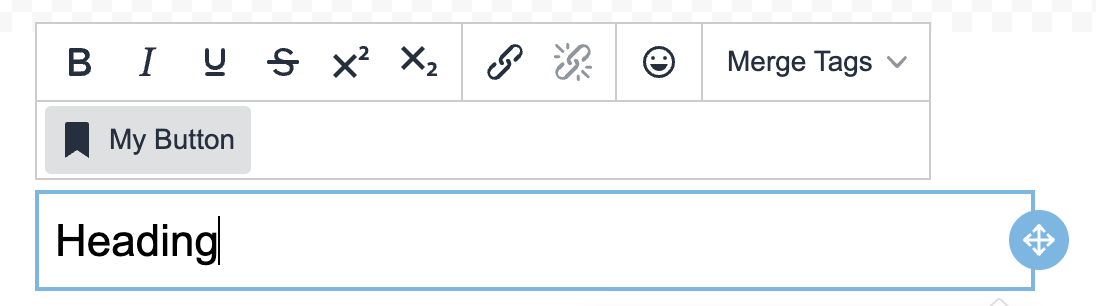
unlayer.init({
features: {
textEditor: {
customButtons: [
{
name: 'my_button',
text: 'My Button',
icon: 'bookmark',
onSetup: () => {},
onAction: (data, callback) => {
console.log(data.text);
callback(data.text + ' Updated');
},
},
],
},
},
});
Preheader Text
If you are using the editor in displayMode
email, users can set a preheader. A preheader is the short summary text that follows the subject line when viewing an email from the inbox.
By default, preheader text is turned on. If you want to disable it, you can turn it off.
unlayer.init({
features: {
preheaderText: false
}
})
Smart Merge Tags
Smart Merge Tags is a human-friendly version of merge tags to add dynamic content in your designs. With this feature, users are able to easily identify merge tags within highlighted field and they see human-friendly names instead of code.
By default, smart merge tags are enabled. If you want to disable it, you can turn it off.
unlayer.init({
features: {
smartMergeTags: false
}
})
SVG Images
If you would like to allow SVG image uploads, you can use the following feature flag. By default, SVG image uploads are disabled.
unlayer.init({
features: {
svgImageUpload: true
}
})
Send Test Email
If you would like users to be able to receive a test email in their inbox, you can enable the Send Test Email button. Learn More
unlayer.init({
features: {
sendTestEmail: true
}
})
Updated 6 months ago